By using this webservice we can get the user details based on username. For that first create one new web application
Open visual studio ---> Select File ---> New ---> Web Site ---> select ASP.NET Web Site
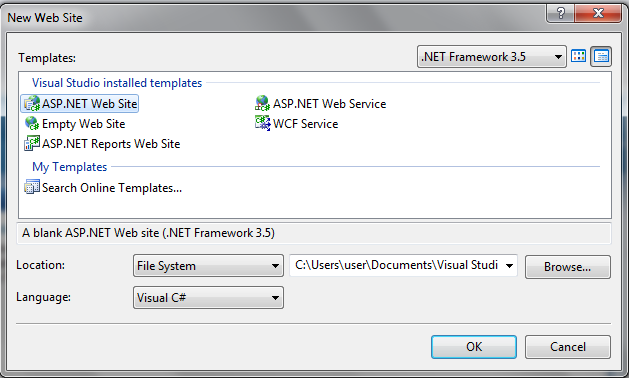
After creation of new website right click on solution explorer and choose “Add web reference” that would be like this
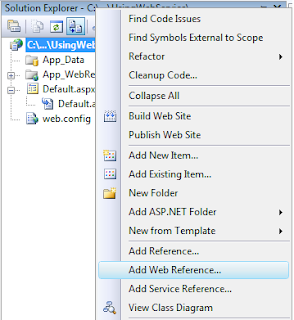
After select Add Web reference option one window will open like this
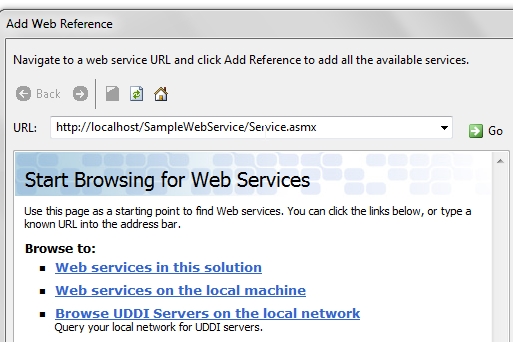
Now
enter your locally deployed web service link and click Go button after
that your web service will found and window will looks like this
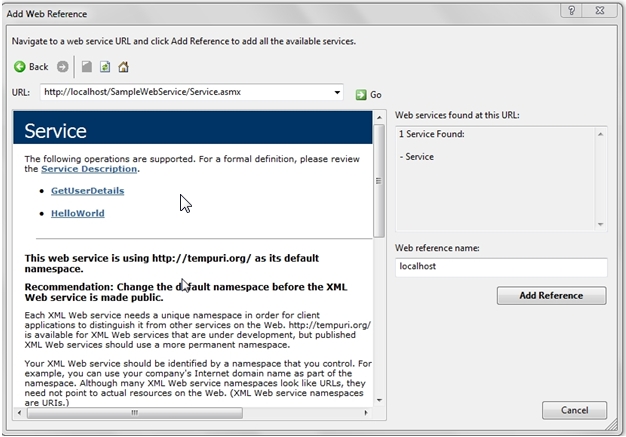
Now click on Add Reference button web service will add successfully. Now open your Default.aspx page and design like this
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Getting Data from WebService</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<table>
<tr>
<td>
<b>Enter UserName:</b>
</td>
<td>
<asp:TextBox ID="txtUserName" runat="server"></asp:TextBox>
</td>
<td>
<asp:Button ID="btnSubmit" runat="server" Text="Submit" onclick="btnSubmit_Click" />
</td>
</tr>
</table>
</div>
<div>
<asp:GridView ID="gvUserDetails" runat="server" EmptyDataText="No Record Found">
<RowStyle BackColor="#EFF3FB" />
<FooterStyle BackColor="#507CD1" Font-Bold="True" ForeColor="White" />
<PagerStyle BackColor="#2461BF" ForeColor="White" HorizontalAlign="Center" />
<HeaderStyle BackColor="#507CD1" Font-Bold="True" ForeColor="White" />
<AlternatingRowStyle BackColor="White" />
</asp:GridView>
</div>
</form>
</body>
</html>
Now in code behind add following namespaces
using System.Data;
using System.Xml;After adding namespaces write the following code in code behind
protected void Page_Load(object sender, EventArgs e)
{
if(!IsPostBack)
{
BindUserDetails("");
}
}
protected void BindUserDetails(string userName)
{
localhost.Service objUserDetails = new localhost.Service();
// you
need to create the object of the web service
DataSet dsresult = new DataSet();
XmlElement exelement = objUserDetails.GetUserDetails(userName);
if(exelement!=null)
{
XmlNodeReader nodereader = new XmlNodeReader(exelement);
dsresult.ReadXml(nodereader, XmlReadMode.Auto);
gvUserDetails.DataSource = dsresult;
gvUserDetails.DataBind();
}
else
{
gvUserDetails.DataSource = null;
gvUserDetails.DataBind();
}
}
protected void btnSubmit_Click(object sender, EventArgs e)
{
BindUserDetails(txtUserName.Text);
}
Now run your application and check output .When you type R, it will display all the student name that start with the letter R.
No comments:
Post a Comment